Design Booking Service
AirBnB
Functional Requirements
Hotels
- onboarding
- updates
- bookings
User
- Search
- Book
- Check Bookings
Non Functional Requirements
- Low Latency
- High Availability
- High Consistency
Architecture
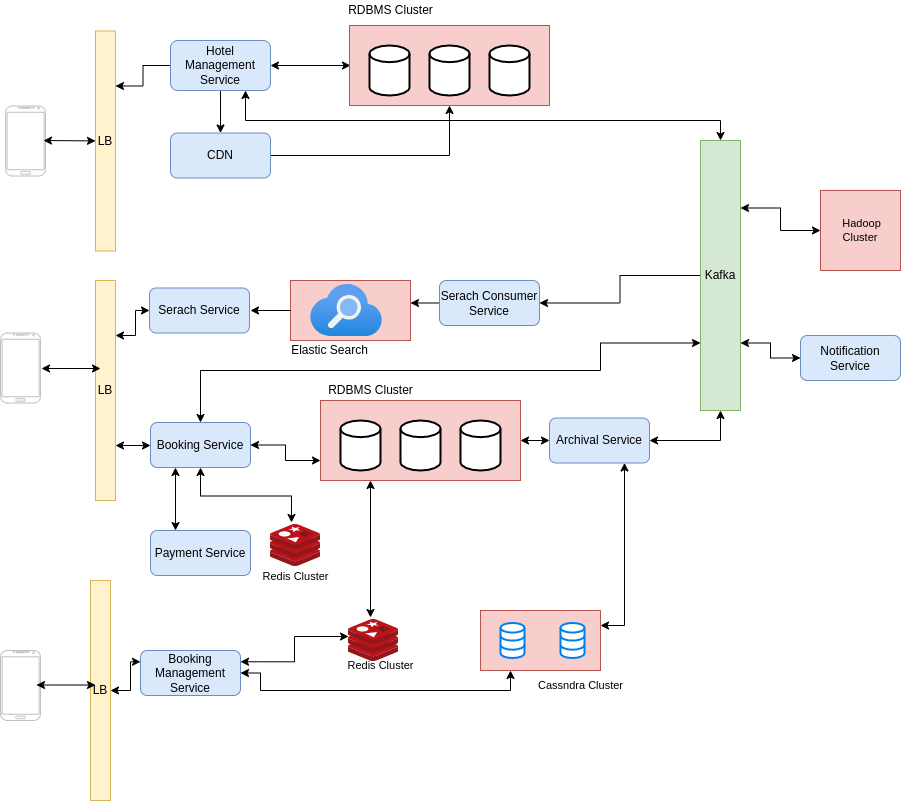
Hotel Management
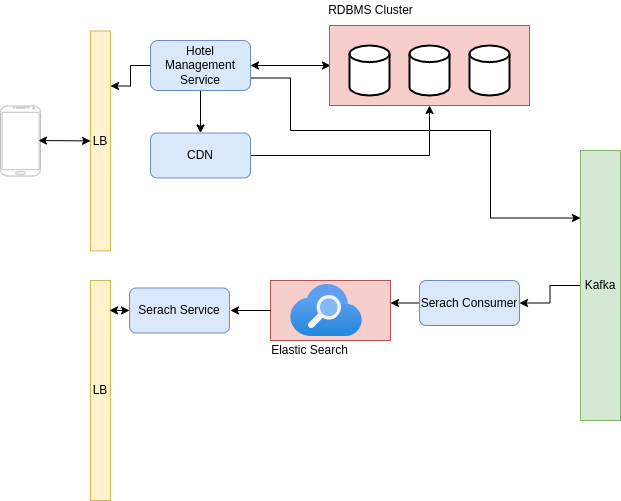
Hotel Service
- Hotels can register themselves on the platform using hotel service
- The hotel data is highly related. It can be stored in an RDBMS cluster
- Media-related information like pictures can be stored in CDN. The URL for the CDN can be stored in the RDBMS database
The updates by the hotel service are sent to the Kafka queue. For example, if a room is added to a hotel, the update is sent to Kafka, where consumers consume that message. One of the consumers is Search Consumer
service.
Serch Consumer Service
- it consumes messages from Kafka queue regarding updates from the hotel service
- pushes the messages from Kafka to Elastic Seach Cluster
Search Service
- Search service sits on top of Elastic Search Cluster
- provides an interface for users to search the hotels
Customer
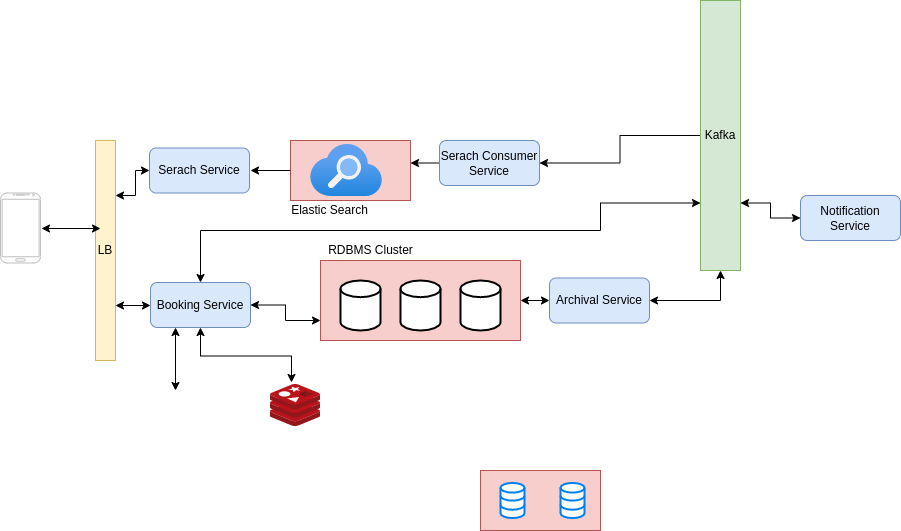
Booking Service
- Sits on top of RDBMS database
- Interacts with Payment service for payments related operations
- the booking messages are sent to Kafka, which are picked up by Search Consumer services and Elastic Search
- When a user books a room, it should not be visible to other users
- When the booking is completed by the user, it is notified to Kafka
-
Service Consumer Service
consumes that message and updates theElastic Search Cluster
and removes the availability of the room - When the user queries
Search Service
, the booked room will not be listed in the availability list
Archival Service
- The bookings that have been completed are moved out of RDBMS and stored in the
Cassandra Cluster
Notification Service
- It provides notification to the users based on the booking operation for eg:
canceled
,booked
,payment success
, etc.
Booking Management Service
- Provides information about the booking history, status
APIs
-
POST /hotel
- add hotel to db
-
GET /hotel/id
- retrieve hotels from db
-
PUT /hotel/id
- update hotel in db
-
PUT /hotel/id/rooms/id
- insert rooms for hotel in db
DB Schema
1. Hotel DB
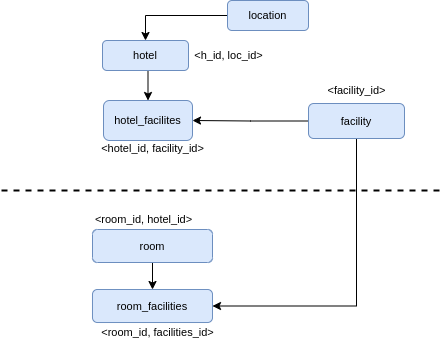
- hotel \(\rightarrow\) [ hotel_id, name, locality_id, description, original_images, display_images, is_active ]
- hotel_facilities \(\rightarrow\) [ id, hostel_id, facility_id, is_active ]
- locality \(\rightarrow\) [ locality_id, city_id, state_id, zipcode, is_active ]
- rooms \(\rightarrow\) [ room_id, hotel_id, display_name, is_active, quantity, price_min, price_max ]
- rooms_facilities \(\rightarrow\) [ id, room_id, facility_id, is_active ]
- facilities \(\rightarrow\) [id, display_name ]
2. Booking DB
- available_rooms \(\rightarrow\) [ room_id, date, initial_qty, available_qty]
- booking_id \(\rightarrow\) [booking_id, room_id, user_id, start_data, end_date, no_of_rooms, status, invoice_id]
- status \(\rightarrow\) [Reserved, Booked, Cancelled, Completed]
Movie Ticket Booking system
enum SeatType {
AC,
NON_AC,
LOUNGE
};
class Theater{
std::vector<Screen> screens;
City city;
int theater_id;
};
class Screen {
int screen_id;
int total_seats;
std::vector<Seats> seats;
};
class Seats {
int seat_no;
SeatType type;
};
class Screening {
int screen_id;
int movie_id;
TimeSlot slot;
};
class Movie {
int movie_id;
std::string description;
};
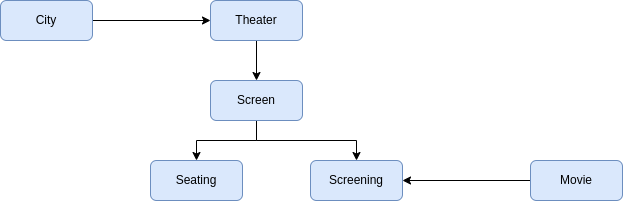
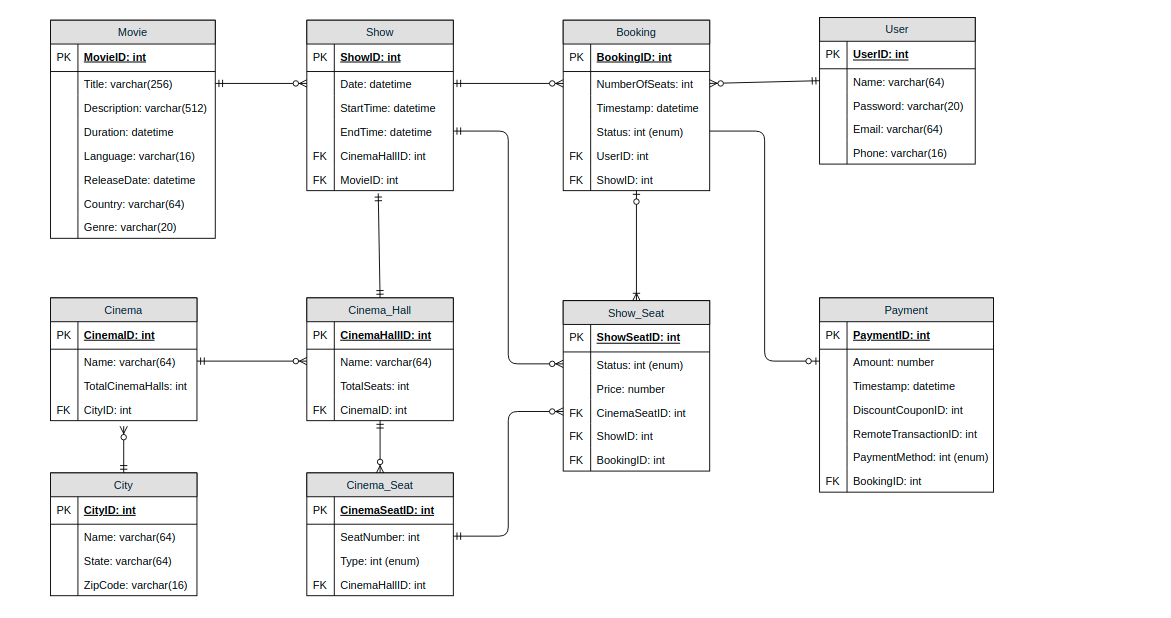